10 Easy Steps to Implement Integration Testing
- Kishan Mehta
- Aug 12, 2024
- 8 min read
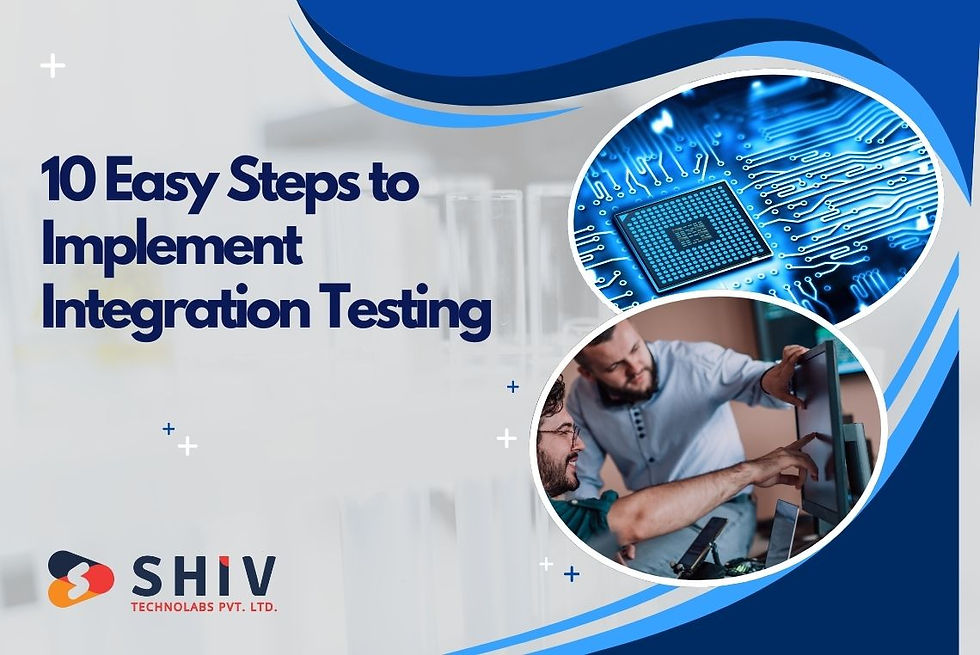
In today’s fast-paced business environment, ensuring that software systems work correctly together is crucial. Integration testing is a key process in the software development lifecycle, allowing businesses to identify issues between integrated systems and ensure a smooth operation. This blog will guide you through the basics of integration testing, including its types, steps for implementation, and specific examples in Laravel.
What is Integration Testing?

Integration testing is a critical phase in software development where different components or systems are tested together as a unified system. Unlike unit testing, which focuses on individual components, integration testing evaluates the interactions between modules to verify they work as intended when combined. This process is essential for web development projects, as it helps identify issues that may arise from the interaction between different parts of the system, such as data flow problems or interface mismatches. The goal is to catch any issues early, ensuring that the complete system functions correctly and meets user expectations.
Types of Integration Testing
Integration testing can be approached in various ways, depending on the complexity of the system and the specific needs of the business. Here are some common types:
Big Bang Integration Testing: This approach involves integrating all components or modules at once and testing them as a whole. It can be efficient but may lead to challenges in pinpointing the source of any issues.
Incremental Integration Testing: Components are integrated one at a time, and testing is conducted after each integration. This method allows for more manageable testing and easier identification of issues.
Top-Down Integration Testing: Testing starts from the top level of the application and proceeds downward. This method often involves stubs to simulate lower-level modules that are not yet integrated.
Bottom-Up Integration Testing: This approach begins with testing lower-level components and gradually integrates higher-level modules. Drivers are used to simulate higher-level modules during testing.
Sandwich Integration Testing: Combining top-down and bottom-up approaches, this method tests both high and low-level components simultaneously. It can be useful for complex systems where both approaches offer advantages.
Steps to Implement Integration Testing
Implementing integration testing effectively is crucial to ensure that the different components of a software system interact correctly. Here’s a detailed breakdown of each step to guide you through the process:
1. Define Integration Points
Identify Critical Integration Points: Start by mapping out where different modules or components of your system interact. These integration points are where data flows between components, or where one module depends on another. This could include APIs, databases, third-party services, or user interfaces.
Analyze Data Flow and Dependencies: Understand how data moves through the system and identify dependencies between modules. This analysis helps in designing test cases that cover all necessary interactions.
Establish Test Objectives: Clearly define what you aim to achieve with integration testing. This might include verifying data consistency, ensuring smooth communication between modules, or validating that the system meets business requirements.
2. Create Test Cases
Develop Comprehensive Test Scenarios: Based on the integration points identified, create test cases that cover various scenarios. Include both typical and edge cases to ensure thorough testing. For instance, if testing a payment system, consider scenarios like successful transactions, failed payments, and network issues.
Define Expected Results: For each test case, outline the expected outcomes. This will help in assessing whether the integration works as intended or if there are discrepancies that need to be addressed.
Prioritize Test Cases: Prioritize test cases based on their impact on the system and business operations. Critical integration points should be tested first to ensure core functionalities are working correctly.
3. Prepare Test Data
Generate Realistic Test Data: Create test data that closely resembles real-world scenarios. This includes varying data inputs, edge cases, and large data sets. The aim is to simulate actual usage as closely as possible.
Set Up Test Data Repositories: Store test data in a way that it can be easily accessed and managed. This might involve setting up databases, files, or mock services that reflect the production environment.
Ensure Data Privacy and Security: When using real data, ensure that it complies with privacy regulations and does not expose sensitive information. Use anonymized or synthetic data where possible to avoid privacy issues.
4. Set Up the Testing Environment
Replicate the Production Environment: Configure the testing environment to match the production setup as closely as possible. This includes setting up servers, databases, and network configurations to reflect the live system.
Install Required Tools and Frameworks: Ensure that all necessary testing tools, frameworks, and libraries are installed and properly configured. This might include testing frameworks, continuous integration tools, or environment-specific configurations.
Establish Access and Permissions: Make sure that the testing environment has the correct access controls and permissions to interact with all necessary components. This ensures that tests can be run without encountering permission issues.
5. Execute Tests
Run Integration Tests: Execute the test cases according to the predefined scenarios. Ensure that each test is conducted in a controlled environment to accurately capture results.
Monitor System Behavior: While running tests, closely observe the system’s behavior for any anomalies or failures. This includes checking logs, system performance, and user interface responses.
Record Test Results: Document the results of each test, including any errors or unexpected behaviors. Accurate recording helps in analyzing issues and tracking the progress of integration testing.
6. Document Results
Compile Test Reports: Create detailed reports that summarize the results of the integration tests. Include information such as test cases executed, outcomes, defects found, and any deviations from expected results.
Track Defects: Log any defects or issues identified during testing. Include details about the nature of the defect, steps to reproduce it, and its impact on the system.
Provide Feedback: Share the test results and feedback with the development team to facilitate prompt resolution of issues. Clear communication helps in addressing problems efficiently and improving the overall system.
7. Analyze and Fix Issues
Identify Root Causes: Analyze the defects or issues identified during testing to determine their root causes. This involves examining code, configurations, and interactions between components.
Collaborate with Development Team: Work closely with the development team to address the identified issues. Provide detailed information about the defects to assist in the debugging and fixing process.
Verify Fixes: Once issues are resolved, retest the affected components to confirm that the fixes are effective and that no new issues have been introduced.
8. Re-Test
Run Tests Again: After fixes are implemented, re-run the integration tests to ensure that the issues have been resolved and that the system functions as expected.
Verify Comprehensive Coverage: Ensure that the re-testing covers all relevant scenarios, including those that were previously problematic. This helps in confirming that the issues have been fully addressed.
Update Test Cases: Modify or add new test cases if needed to reflect any changes made during the fixing process. This ensures that future testing remains thorough and relevant.
9. Automate Tests
Select Automation Tools: Choose appropriate automation tools or frameworks that fit your integration testing needs. These tools can help streamline the testing process and improve efficiency.
Implement Automated Test Scripts: Develop and implement automated test scripts based on the previously defined test cases. Automation helps in running repetitive tests and integrating testing into the CI/CD pipeline.
Maintain Test Automation: Regularly update and maintain automated tests to keep them aligned with changes in the system. Automated tests should be reviewed and refined as the application evolves.
10. Review and Improve
Conduct Test Reviews: Periodically review the integration testing process to identify areas for improvement. This includes evaluating test coverage, effectiveness, and efficiency.
Incorporate Feedback: Gather feedback from stakeholders, including developers, testers, and business users, to improve the integration testing process. Implement changes based on this feedback to enhance testing practices.
Adapt to Changes: Stay updated with changes in technology, tools, and best practices. Adjust the integration testing approach as needed to adapt to new developments and ensure continuous improvement.
Integration Testing in Laravel
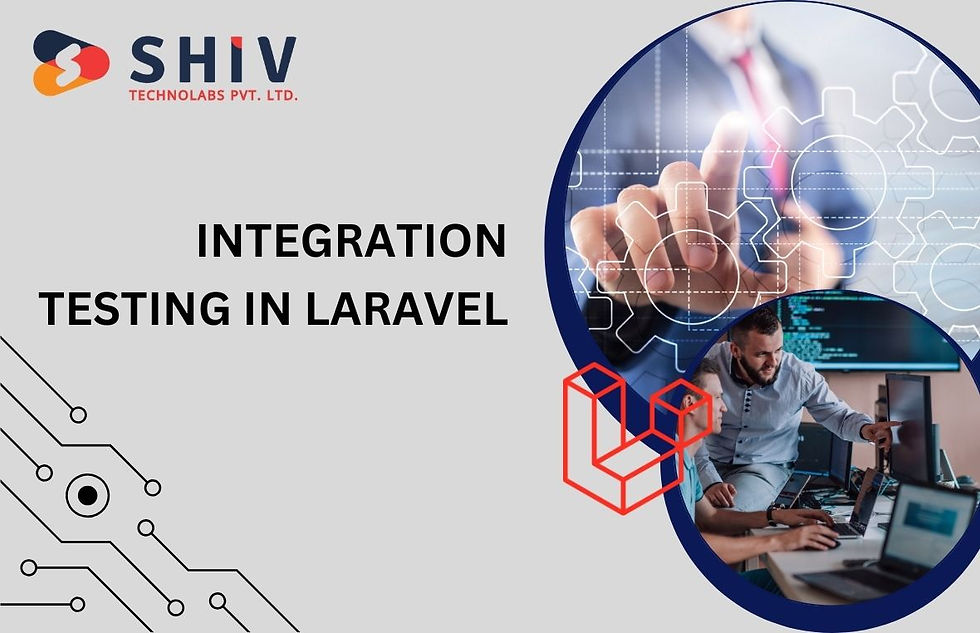
Laravel offers a range of features and tools that facilitate integration testing, making it an excellent choice for businesses seeking efficient Laravel development solutions. These tools help streamline the testing process and ensure that components work together as expected. Here are some key features:
Built-In Testing Framework: Laravel includes a built-in testing framework based on PHPUnit, which is a widely used tool for writing and running tests in PHP. This framework supports both unit and integration testing.
Test Suites: Laravel allows you to organize tests into different suites, making it easier to manage and execute tests based on their type or purpose.
Mocking and Stubbing: Laravel provides support for mocking and stubbing dependencies, which helps simulate interactions between components without relying on real implementations. This is useful for testing integrations in isolation.
Database Migrations and Seeders: Laravel’s migration system allows you to create and manage database schemas, while seeders populate the database with test data. This ensures that the testing environment mirrors the production setup.
HTTP Testing: Laravel includes tools for simulating HTTP requests and responses, enabling you to test the integration of web routes and controllers. This helps verify that API endpoints and web interfaces work correctly.
Testing Helpers: Laravel offers various helper methods for interacting with the application during tests. These helpers simplify tasks such as database assertions, form submissions, and response checks.
Artisan Command for Testing: The Artisan command-line tool includes commands specifically for running tests, managing test environments, and generating test stubs, which helps streamline the testing workflow.
Environment Configuration: Laravel supports environment-specific configurations, allowing you to set up different testing environments. This includes configuring database connections, mail drivers, and other services used during testing.
Test Case Generation: Laravel provides commands to generate test cases, making it easier to create and organize tests for your application’s components and integrations.
Integration with CI/CD Pipelines: Laravel’s testing features can be integrated into continuous integration and continuous deployment (CI/CD) pipelines, allowing automated testing as part of the deployment process.
Assertions and Verification: Laravel’s testing framework includes a range of assertions to verify the correctness of responses, data, and application behavior. This helps ensure that integrations meet expected outcomes.
Database Transactions: Laravel supports testing within database transactions, allowing tests to run in isolation and rollback changes after execution. This maintains a clean state for each test run.
Conclusion
Integration testing is a crucial process for ensuring that different components of a software system work together as expected. By systematically defining integration points, creating comprehensive test cases, preparing realistic test data, setting up a robust testing environment, and executing tests, businesses can identify and address issues effectively. The steps outlined above not only help in validating the interactions between modules but also in maintaining the overall reliability and performance of the system.
Integration testing in Laravel offers powerful tools and features that simplify the process, making it easier to test complex interactions and ensure that your application functions smoothly. By leveraging Laravel’s built-in testing framework, mocking capabilities, and support for HTTP testing, you can enhance your testing efforts and deliver high-quality software.
If you’re looking for a trusted partner to handle your integration testing needs and more, Shiv Technolabs is here to help. As a leading Laravel development company in Saudi Arabia, we specialize in providing top-notch development and testing services to ensure your applications perform at their best. Our team of experienced professionals is dedicated to delivering solutions that meet your business needs and exceed your expectations. Contact us today to learn how we can support your next project with our expert Laravel development services.
Comments