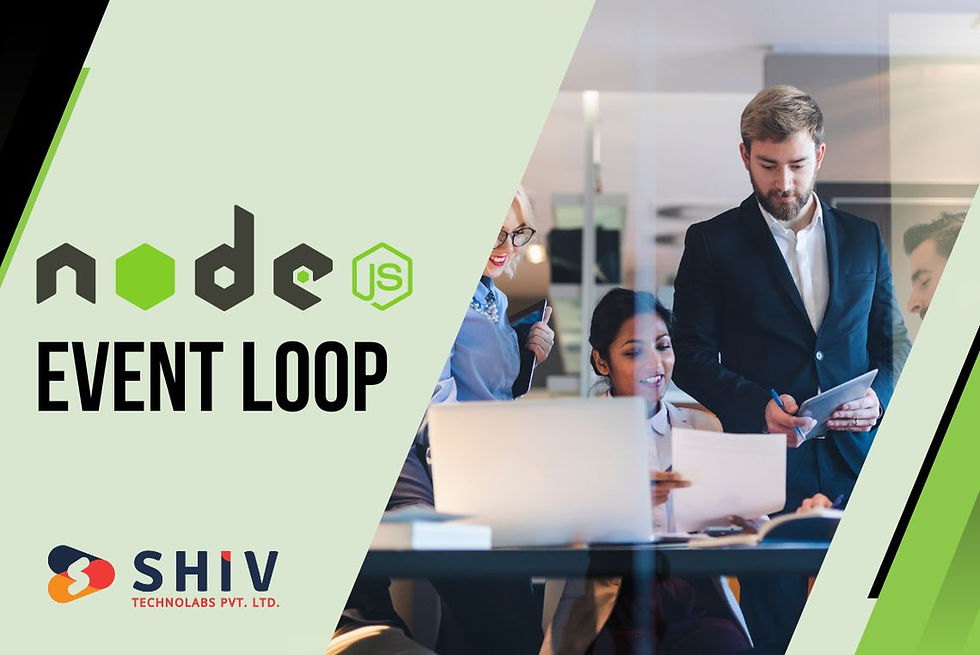
Node.js stands out as a powerful tool for building scalable network applications. A key aspect of Node.js is its event-driven architecture, which is driven by the Node.js event loop. For developers in Australia, understanding the intricacies of the Node.js event loop is crucial for writing efficient code and building robust applications.
This guide will delve into the Node.js event loop, breaking it down and offering insights on how it operates.
Introduction to Node.js Event Loop
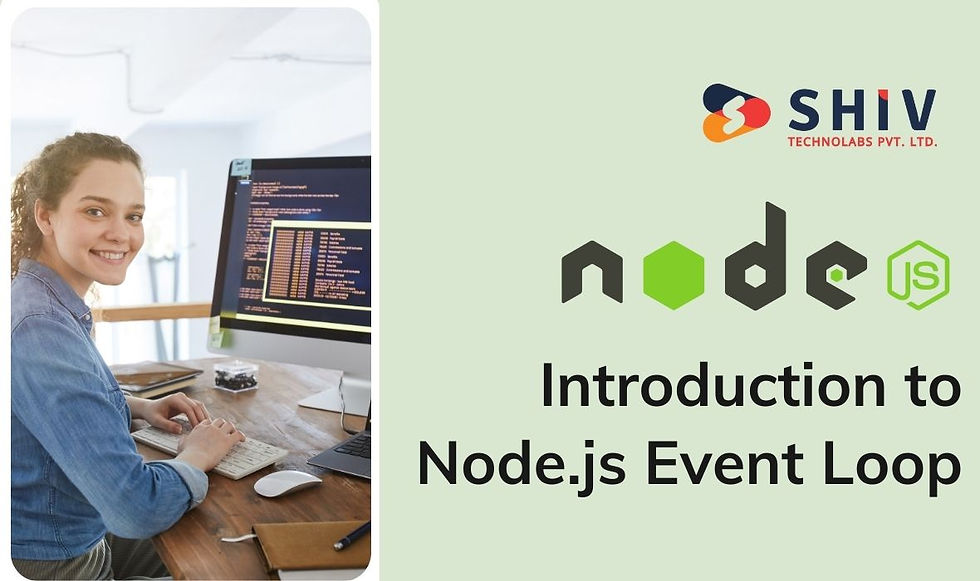
The Node.js event loop is fundamental to the way Node.js handles asynchronous operations. It is a single-threaded mechanism that manages multiple tasks by utilizing non-blocking I/O operations. This design allows Node.js to handle numerous connections simultaneously without needing to spawn multiple threads.
To get started, it’s important to understand that Node.js uses an event-driven model. This means that the core of Node.js is built around the concept of events and listeners. The event loop processes these events and executes the corresponding callbacks, enabling Node.js to perform non-blocking operations efficiently.
How the Node.js Event Loop Works
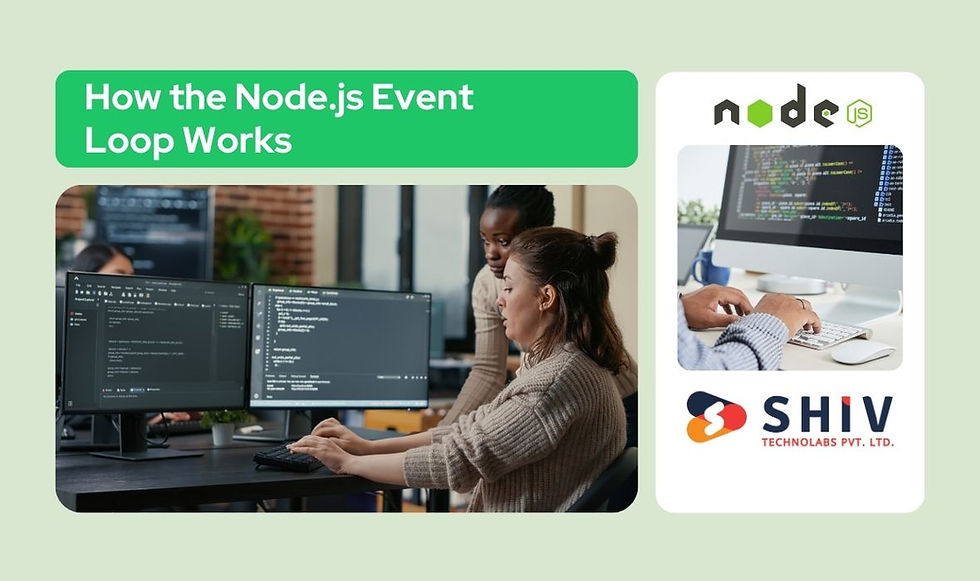
The Node.js event loop is a core component that allows Node.js to perform non-blocking I/O operations efficiently despite being single-threaded. Understanding how the event loop operates is crucial for developers looking to build high-performance applications with Node.js. Here's a detailed look at how the Node.js event loop works:
1. Initialization
When a Node.js application starts, the runtime initializes the event loop. This involves setting up the environment and preparing the event loop to start processing tasks. The event loop is a key part of the Node.js runtime that handles asynchronous operations and ensures that your application remains responsive.
2. Event Loop Phases
The Node.js event loop operates through a series of phases, each responsible for handling different types of operations. The event loop continuously cycles through these phases, executing callbacks and managing tasks. Here’s a breakdown of each phase:
Timers Phase
Purpose: Executes callbacks scheduled by setTimeout() and setInterval().
How It Works: When you set a timer using setTimeout() or setInterval(), the callback is added to the timers queue. The event loop checks this queue and executes the callbacks once the specified time has elapsed.
I/O Callbacks Phase
Purpose: Handles callbacks for I/O operations such as reading files, network requests, and database queries.
How It Works: After the timers phase, the event loop processes I/O callbacks that were deferred in previous phases. This ensures that I/O operations complete and their results are handled.
Idle, Prepare Phase
Purpose: This phase is used for internal operations and is not directly interacted with by developers.
How It Works: It is mainly used for preparatory tasks and ensuring that the event loop can handle new events efficiently.
Poll Phase
Purpose: Retrieves new I/O events and executes their callbacks.
How It Works: The poll phase is where the event loop waits for new events. If there are any pending I/O operations, their callbacks are executed. If there are no events, the event loop can either wait for a new event or proceed to the next phase.
Check Phase
Purpose: Executes callbacks scheduled by setImmediate().
How It Works: Callbacks added via setImmediate() are processed in this phase. Unlike timers, which are scheduled based on time, setImmediate() schedules callbacks to be executed immediately after the current event loop cycle.
Close Callbacks Phase
Purpose: Handles callbacks for closing operations such as socket.on('close').
How It Works: This phase processes callbacks that are triggered when resources are closed. For example, when a network connection or file stream is closed, associated callbacks are executed here.
3. Event Loop Execution Flow
Here’s a simplified flow of how the Node.js event loop processes tasks:
Start Event Loop: When the application begins, the event loop starts and begins processing events and callbacks.
Execute Timers: The event loop checks the timers queue and executes any callbacks that are due.
Process I/O Callbacks: Handles callbacks for I/O operations that were deferred in earlier phases.
Idle, Prepare: Performs internal tasks and prepares for the next cycle.
Poll Events: Waits for new I/O events and processes their callbacks. If no events are present, it waits or continues to the next phase.
Execute Immediate Callbacks: Processes callbacks scheduled by setImmediate().
Handle Close Callbacks: Executes any callbacks related to closing operations.
Repeat: The event loop continues to cycle through these phases, processing tasks and events as they come.
Node.js Event Loop Explained with Examples
To better understand the Node.js event loop, consider the following example of asynchronous operations:
javascript
console.log('Start');
setTimeout(() => {
console.log('Timeout 1');
}, 1000);
setTimeout(() => {
console.log('Timeout 2');
}, 0);
console.log('End');
In this code:
The console.log('Start') executes immediately.
The setTimeout(() => { console.log('Timeout 1'); }, 1000); schedules a callback to run after 1000 milliseconds.
The setTimeout(() => { console.log('Timeout 2'); }, 0); schedules a callback to run as soon as possible.
Finally, console.log('End') executes before the timeout callbacks.
The output will be:
sql
Start
End
Timeout 2
Timeout 1
This demonstrates how the event loop prioritizes callbacks based on their timing and phase.
Using For Loops in Node.js
When writing code in Node.js, you might come across scenarios where you use loops. Understanding how these loops interact with the Node.js event loop is crucial. Here’s an example using a for loop:
javascript
console.log('Start')
for (let i = 0; i < 3; i++) {
setTimeout(() => {
console.log(`Timeout ${i}`);
}, 1000);
}
console.log('End');
In this code:
The for loop schedules three timeouts with a delay of 1000 milliseconds each.
console.log('End') is executed before the timeouts, but all timeouts will be processed after the event loop has reached the timers phase.
The output will be:
sql
Start
End
Timeout 0
Timeout 1
Timeout 2
This shows that even though the for loop schedules multiple timeouts, they are still subject to the event loop’s phases and timing.
Practical Applications and Considerations
For Australian developers working with Node.js, understanding the event loop is essential for creating responsive applications. Here are a few practical considerations:
Asynchronous Programming: Use asynchronous functions and callbacks to avoid blocking the event loop. This ensures that your application remains responsive.
Error Handling: Ensure that errors are properly handled in asynchronous callbacks to prevent unhandled exceptions from disrupting the event loop.
Performance Optimization: While the Node.js event loop is efficient, it’s important to avoid long-running operations within the event loop. Offload CPU-intensive tasks to worker threads or external services when necessary.
Testing and Debugging: Use tools and techniques to monitor and debug event loop performance. This can help in identifying bottlenecks and optimizing your Node.js applications.
Conclusion
Mastering the Node.js event loop is crucial for any developer working with Node.js. Understanding how it manages asynchronous operations and how it interacts with various programming constructs, like for loops, is essential for writing efficient and effective code. For those engaged in Node.js development in Australia, leveraging the expertise of local Node.js developers can further enhance your projects and ensure they meet the highest standards.
By grasping the intricacies of the Node.js event loop, you can optimize your applications and make the most of Node.js’s powerful features. If you’re seeking to deepen your understanding of Node.js or need assistance with Node.js development, numerous companies in Australia specialize in this field. Whether you’re looking for a Node.js development company in Australia or are interested in working with experienced Node.js developers in Australia, there are many options available. These professionals can offer tailored solutions and expertise to help you leverage the full potential of Node.js for your projects.
Comments